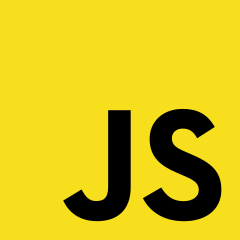
Javascript – Play with strings and arrays
Last weekend I was playing with strings in Javascript and I made different exercises with them, so this is a good moment to write a remember post with this simple commands.
Exercise 1: Discard first n characters of a string, in this case 3
var $myString = ("Hello world"); var $myStringCut = (($myString).substring(3)); //Output will be "lo world";
Exercise 2: Get the string until certain character, in this case we will get all the characters before “&”
var $myString = ("Hello world&Hello People"); var $myStringCut= $myString.substr(0, $myString.indexOf('&')); //Output will be "Hello World"
Exercise 3: Get the string after certain character, in this case we will get all after “&” skipping first 5 characters
var $myString = ("Hello world&xdftHello People"); var $myStringCut = ($myString.substring($myString.indexOf('&')+5)) ; //Output will be "Hello People"
Exercise 4: Select character in certain position. How to select the third character, take in mind we have to start counting first element with 0, for this reason 2 will be the third.
var $myString = ("Hello world"); var $myStringCut = ($myString.substr(2, 1)); //Output will be "l"
Exercise 5: Convert string into array, In this case we will split a string into array using existing spaces as separators ” ”
var $myString = ("Hello world Hello People"); var $array = $myString.split(' '); //Output will be "Hello,world,Hello,People"
Exercise 6: Select element inside Array, in this case we will select the second element.
var $myString = ("Hello world Hello People"); var $array = $myString.split(' '); //Array will be "Hello,world,Hello,People" var $array2nElement = String($array.slice(1,2)); //Output will be "world"
Exercise 7: Select 2 element inside Array, in this case we will select the second and the third element.
var $myString = ("Hello world Hello People"); var $array = $myString.split(' '); //Array will be "Hello,world,Hello,People" var $array2nElement = String($array.slice(1,3)); //Output will be "world,Hello"
Exercice 8: Create an array splitting every charecter
var $myString = ("abcdefg"); var $array = $myString.split(''); //Output will be "a,b,c,d,e,f,g"
Exercise 9: Split string into array, convert elements into integers and sum all the items
var $myString = ("12345"); //Split our array var $array = $myString.split(''); //Convert array element into a Int characters var $arrayNum=[]; for (var i=0,l=$array.length;i<l;i++) $arrayNum.push(+$array[i]); //sum all numbers var $sum = $arrayNum.reduce(add, 0); //Output will be 15 function add(a, b) { return a + b; }
Exercise 10: Capitalize or Decapitalize an existing string. Sometimes you want to avoid case sensitive problems and a good way to do that is capitalize or decapitalize stings
var $myString = "Hello World!"; var $myStringUpper = $myString.toUpperCase(); //Output will be "HELLO WORLD"
var $myString = "Hello World!"; var $myStringUpper = $myString.toLowerCase(); //Output will be "hello world"